Model 3: The UTC Wall Clock¶
Date: | 2017-09-18 |
---|
Design Decisions¶
- 128 ticks/sec, generated from external RTC clock (32768 Hz)
- uptime counter
- multitasker
- a battery backed real time clock is connected via i2c
- start time is read from RTC
- new: RTC generates 32768 Hz signal to drive clock ticks
- new: run epoch seconds as additional software clock
- new: use epoch seconds to derive times in 3 different time zones, read desired zone from 2 input pins
- new: conversion to and from epoch seconds: additional
.short
versions use cached values for some epoch, e.g. 2017- new: shift register drives 7-segment digits as display
Description¶
The code included below is a complete, working example, tested on an
atmega644p controller. The syntax for the includes is such that
amforth-shell.py
will upload the programm and resolve all
#include file
directives.
This clock is a more traditional design with 4 large 7-Segment digits and a good RTC (battery backed). Placed in a nice housing it can be used standalone.
The Pinout section should be familiar by now. Using quotations
([: ... ;]
) the LED definitions can have alias names, which might
be more useful in the given context.
The Display is driven by shift registers as before. These are connected to 7-segment digits, not individual LEDs.
Time zones are selected by reading 2 pins.
The Real Time Clock is a different chip (DS3231). It needs somewhat adapted functions to read and set the time counters. The chip is much more accurate than the clock sources I have used before.
The counters of the master clock are unchanged, uptime is counted as before. The source of the clock tick has changed. The 32768 Hz square wave signal is driving timer/counter0 which overflows 128 times per second. The corresponding interrupt service routine increments a counter, the main loop checks whether a half second has passed.
Functions to set/read/display the counters of the master clock are available. Functions to copy time counter values from between the master clock and the RTC follow.
Handling of time zones, epoch seconds, and the display of a local time are handled as described in section Time Zones.
Multitasking, periodic jobs, a background task to run the main loop of the master clock — everything is as described before (Model 2).
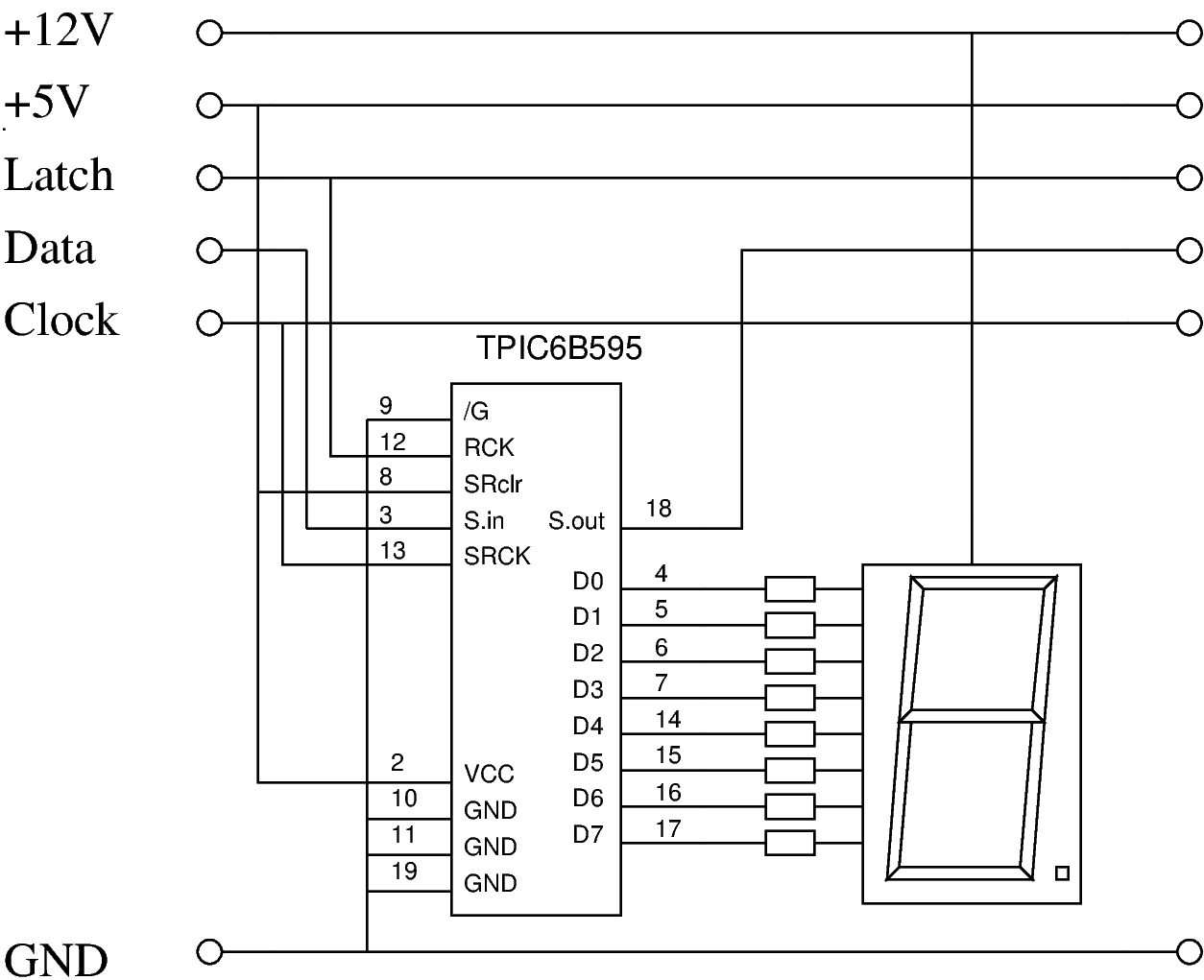
Schematic for one 7-segment digit
The Code¶
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 194 195 196 197 198 199 200 201 202 203 204 205 206 207 208 209 210 211 212 213 214 215 216 217 218 219 220 221 222 223 224 225 226 227 228 229 230 231 232 233 234 235 236 237 238 239 240 241 242 243 244 245 246 247 248 249 250 251 252 253 254 255 256 257 258 259 260 261 262 263 264 265 266 267 268 269 270 271 272 273 274 275 276 277 278 279 280 281 282 283 284 285 286 287 288 289 290 291 292 293 294 295 296 297 298 299 300 301 302 303 304 305 306 307 308 309 310 311 312 313 314 315 316 317 318 319 320 321 322 323 324 325 326 327 328 329 330 331 332 333 334 335 336 337 338 339 340 341 342 343 344 345 346 347 348 349 350 351 352 353 354 355 356 357 358 359 360 361 362 363 364 365 366 367 368 369 370 371 372 | \ 2017-08-30 main-20-utc-wallclock.fs
\
\ Written in 2017 by Erich Wälde <erich.waelde@forth-ev.de>
\
\ To the extent possible under law, the author(s) have dedicated
\ all copyright and related and neighboring rights to this software
\ to the public domain worldwide. This software is distributed
\ without any warranty.
\
\ You should have received a copy of the CC0 Public Domain
\ Dedication along with this software. If not, see
\ <http://creativecommons.org/publicdomain/zero/1.0/>.
\
\ include syntax for upload with amforth-shell.py
\
\ 11.059200 MHz main crystal
\ 32768 Hz square signal on pin T0
\ timer/counter0
\ 128 ticks/second
\
\ minimal clock
\ plus i2c, i2c RTC (ds3231)
\ display: shift registers (TPIC 6B595) and 4 7-segment digits
\ MasterClock in UTC, display in 2 other timezones
\ 2 pins for selection of timezone
#include erase.frt
#include bitnames.frt
#include marker.frt
\ these definitions are resolved by amforth-shell.py as needed
\ include atmega644p.fs
#include flags.frt
#include 2variable.frt
#include 2constant.frt
#include 2-fetch.frt
#include 2-store.frt
#include m-star-slash.frt
#include quotations.frt
#include avr-defers.frt
#include defers.frt
#include eallot.frt
#include 2evalue.frt
marker --start--
PORTA $03 bitmask: _tz
\ PORTB 0 portpin: T0
\ PORTB 1 portpin: T1
PORTB 2 portpin: led.0
PORTB 3 portpin: led.1
PORTB 4 portpin: led.2
PORTB 5 portpin: led.3
: led_dcf [: led.0 ;] execute ;
: led_utc [: led.1 ;] execute ;
: led_mez [: led.2 ;] execute ;
: led_mesz [: led.3 ;] execute ;
PORTC 0 portpin: i2c_scl
PORTC 1 portpin: i2c_sda
\ abakus/4x7seg display
PORTD 4 portpin: sr_data
PORTD 5 portpin: sr_clock
PORTD 6 portpin: sr_latch
\ --- famous includes and other words
: ms ( n -- ) 0 ?do pause 1ms loop ;
: u0.r ( u n -- ) >r 0 <# r> 0 ?do # loop #> type ;
: odd? ( x -- t/f ) $0001 and 0= 0= ;
: even? ( x -- t/f ) $0001 and 0= ;
\ --- driver: status leds
#include leds.fs
\ --- driver: time zone switch
: +sw ( -- ) _tz pin_input ;
\ --- driver: i2c rtc clock
: bcd>dec ( n.bcd -- n.dec )
$10 /mod #10 * + ;
: dec>bcd ( n.dec -- n.bcd )
#100 mod #10 /mod $10 * + ;
#include i2c-twi-master.frt
#include i2c.frt
#include i2c-detect.frt
: +i2c ( -- )
i2c_scl pin_pullup_on
i2c_sda pin_pullup_on
i2c.prescaler/1
#6 \ bit rate --- 400kHz @ 11.0592 MHz
i2c.init
;
: i2c.scan
base @ hex
$79 $7 do
i i2c.ping? if i 3 .r then
loop
base !
cr
;
$68 constant i2c_addr_rtc
#2000 constant Century
#include i2c_rtc_ds3231.fs
\ --- master clock
\ --- timeup
#include timeup_v0.0.fs
\ tu.counts -- fields available as:
\ tick sec min hour day month year
\ last_day_of_month ( year month -- last_day )
\ timeup.init
\ timeup
\ tu.upd.limits ( Y m -- )
\ --- uptime
2variable uptime
: .uptime ( -- ) uptime 2@ decimal ud. [char] s emit ;
: ++uptime ( -- ) 1. uptime 2@ d+ uptime 2! ;
\ --- timer0 clock tick
\ 128 ticks/sec
\ timer_0_ overflow
\ clock source pin T0 @ 32768 Hz (from ds3231)
#include clock_tick0_external.fs
\ +ticks
\ tick.over? ( -- t/f )
\ tick.over!
\ half.second.over? ( -- 0|1|2 )
: clock.set ( Y m d H M S -- )
sec ! min ! hour !
1- day !
over over
1- month ! year !
( Y m ) tu.upd.limits
;
: clock.get ( -- S M H d m Y )
sec @ min @ hour @
day @ 1+ month @ 1+ year @
;
: clock.dot ( S M H d m Y -- )
#4 u0.r [char] - emit #2 u0.r [char] - emit #2 u0.r [char] _ emit
#2 u0.r [char] : emit #2 u0.r [char] : emit #2 u0.r
;
: clock.show ( -- )
clock.get
clock.dot
;
: .date
year @ 4 u0.r
month @ 1+ 2 u0.r
day @ 1+ 2 u0.r
;
: .time
hour @ 2 u0.r [char] : emit
min @ 2 u0.r [char] : emit
sec @ 2 u0.r
;
: hwclock>clock ( -- )
rtc.get \ -- sec min hour wday day month year
year !
1- month !
1- day !
( wday ) drop
hour !
min !
sec !
year @ month @ 1+ tu.upd.limits
;
: clock>hwclock ( -- )
year @ month @ 1+ day @ 1+
1 \ sunday ":-)
hour @ min @ sec @
( Y m d wday H M S ) rtc.set
;
#include shiftregister.fs
#include 7seg_1.fs
\ --- epoch seconds, timezones
: u>= ( n n -- t/f ) u< invert ;
: d>s ( d -- n ) drop ;
variable _last_epoch
2variable _last_esec
#2017 Evalue EE_last_epoch
#1483228800. 2Evalue EE_last_esec \ 2017
#include epochseconds.fs
2variable Esec
: ++Esec ( -- ) Esec 2@ 1. d+ Esec 2! ;
: .Esec ( -- ) Esec 2@ ud. ;
2variable EsecOffset
: UTC ( -- ) 0. EsecOffset 2! ;
: MEZ ( -- ) 3600. EsecOffset 2! ;
: MESZ ( -- ) 7200. EsecOffset 2! ;
: _tz.set
_tz pin@
dup 0 = if
UTC
led_utc on led_mez off led_mesz off
then
dup 1 = if
MEZ
led_utc off led_mez on led_mesz off
then
dup 2 = if
MESZ
led_utc off led_mez off led_mesz on
then
dup 3 = if
UTC
led_utc on led_mez off led_mesz off
then
drop
;
: local.dt ( -- S M H d m Y )
Esec 2@ EsecOffset 2@ d+ s>dt.short
;
: cd.localtime
local.dt \ -- S M H d m Y
drop drop drop \ -- S M H
rot drop swap \ -- H M
>r #10 /mod swap \ -- H.10 H.1
r> #10 /mod swap \ -- H.10 H.1 M.10 M.1
#4 type.7seg \ --
;
\ --- multitasker
#include multitask.frt
: +tasks multi ;
: -tasks single ;
\ --- timeup jobs ---------------------------
: job.tick
;
: job.sec
++uptime
++Esec
;
: job.min
_tz.set cd.localtime
;
: job.hour ;
: job.day ;
: job.month
\ update length of month in tu.limits
year @ month @ 1+ tu.upd.limits
;
: job.year ;
create Jobs
' job.tick ,
' job.sec , ' job.min , ' job.hour ,
' job.day , ' job.month , ' job.year ,
variable jobCount
: jobCount++
jobCount @
6 < if
1 jobCount +!
then
;
\ --- task 2 --------------------------------
: run-masterclock
['] tx-poll to emit \ add emit to run-masterclock
begin
tick.over? if
tick.over!
1 tick +!
job.tick
then
half.second.over?
dup 0<> if
dup odd? if \ half second
led.1 off
else \ second
led.1 on
timeup
0 tick !
1 jobCount !
then
then
drop
\ run one job per loop, not all at once
jobCount @
bv tu.flags fset?
if
jobCount @ dup
Jobs + @i execute
bv tu.flags fclr
then
jobCount++
pause
again
;
$40 $40 0 task: task-masterclock \ create task space
: start-masterclock
task-masterclock tib>tcb
activate
\ words after this line are run in new task
run-masterclock
;
: starttasker
task-masterclock task-init \ create TCB in RAM
start-masterclock \ activate tasks job
onlytask \ make cmd loop task-1
task-masterclock tib>tcb alsotask \ start task-2
multi \ activate multitasking
;
\ --- main ----------------------------------
: init
+sr
$00 byte>sr $00 byte>sr $00 byte>sr $00 byte>sr
sr_latch low sr_latch high
+sw
+leds leds-intro
#2017 1 1 0 0 0 clock.set
0. uptime 2!
0. Esec 2!
EE_last_epoch _last_epoch !
EE_last_esec _last_esec 2!
+ticks
timeup.init
+i2c
i2c_addr_rtc i2c.ping? if
hwclock>clock
clock.get ut>s.short Esec 2!
else
_last_epoch @ 1 1 0 0 0 clock.set
_last_esec 2@ Esec 2!
then
_tz.set cd.localtime
;
: run
init
starttasker
;
: run-turnkey
applturnkey
init
starttasker
;
\ ' run-turnkey to turnkey
: .d ( -- )
decimal
.uptime space space
clock.show space
tick @ . space
ct.ticks.follow @ . space space
.Esec space
Esec 2@ EsecOffset 2@ d+
s>dt.short clock.dot
cr
;
|